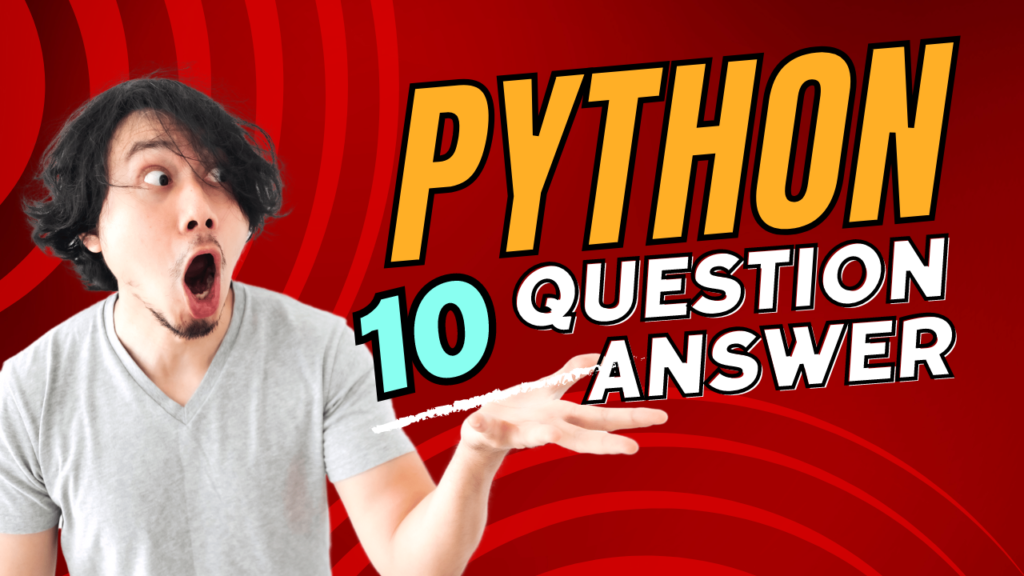
1 Question: – What is Python and Explain it’s Key features?
Python is a high-level and interpreted programming language, which is known for its readability, simplicity and versatility. It was created in 1991 by the Guido van Rossum. Python are more famous as compare to other languages, the Big Reason behind this is – Python supports multiple programming paradigms, including procedural, object oriented, and functional programming style. And the main strength of Python is its extensive standard library, which offers modules and packages for various tasks like file I/O, networking and string manipulation.
Here are the key features of Python,
- Dynamic Typing
- Multi Paradigm
- Rich Standard Library
- Platform Independent
- Extensive Third Party Libraries
- Easy Integration
2 Question: – Explain Python’s Dynamic Typing.
Python Dynamic Typing is a fundamental feature that distinguishes it from the statically typed languages like C or Java. It refers to the capability of Python to determine the type of variable or object at runtime, rather than requiring explicit type declarations in the source code.
This means that developer don’t need to specify the data type of variables when they are declared; instead, Python infers and assign the appropriate type based on the value assigned to the variable.
3 Question: – Difference Between Lists, Tuples, and Dictionaries.
Let’s compare the, Lists, Tuples, and Dictionaries…
Characteristic | List | Tuples | Dictionaries |
---|---|---|---|
Definition | Ordered collection of items. | Ordered, immutable collection of items. | Unordered collection of key-value pairs. |
Mutability | Mutable: can be modified (add, remove, change items) | Immutable: cannot be modified after creation | Mutable: can be modified (add, remove, change items) |
Syntax | Defined with square brackets [ ] | Defined with parentheses ( ) | Defined with curly braces { } |
Accessing Elements | Elements accessed by index (e.g., my_list[0] ) | Elements accessed by index (e.g., my_tuple[0] ) | Elements accessed by key (e.g., my_dict['key'] ) |
Ordered | Preserves order of elements | Preserves order of elements | Does not preserve order of elements (prior to Python 3.7) |
Membership Testing | Supports in operator to check if an item exists in the list | Supports in operator to check if an item exists in the tuple | Supports in operator to check if a key exists in the dictionary |
Usage | Use when you have a collection of items that may change over time (e.g., a list of tasks) | Use when you have a collection of items that should not change (e.g., coordinates of a point) | Use when you need to store key-value pairs for quick look-up (e.g., user details) |
Example | my_list = [1, 2, 3] | my_tuple = (4, 5, 6) | my_dict = {'name': 'John', 'age': 30} |
4 Question: – Difference Between Shallow Copy and Deep Copy in Python.
Here is the difference between Shallow Copy and Deep Copy.
Characteristic | Shallow Copy | Deep Copy |
---|---|---|
Definition | Creates a new object but inserts references to the original nested objects | Creates a new object and recursively copies all nested objects |
Copy Level | Only one level deep: outermost container is duplicated, inner objects remain shared | Recursively duplicates all nested objects to create fully independent copies |
Module | Supported by the copy module (copy.copy() ) | Supported by the copy module (copy.deepcopy() ) |
Usage | Used when you want to duplicate the top-level structure, but share references to nested objects | Used when you need a completely independent copy of all objects, including nested ones |
Performance | Faster and more memory-efficient for large structures with shared components | Slower and consumes more memory due to deep copying of all nested objects |
5 Question: – How do you declare and use variable in Python???
In Python, Declare and using a variable is easy because of it’s dynamic typing and simple syntax. For declare the variable, we just simply assign the value to it using the assignment operator (‘=’). Here is how we declare and used the variabes in Python,
# Variable declaration and assignment my_var = 10 name = "Alice" is_valid = True pi_value = 3.14
6 Question: – Describe the usage of if-else statement in Python.
if-else statements are basically used for conditional execution of the code based on the certain conditions. These statements are allow you to control the flow of your program by specifying different paths or branches to take depending on whether a conditions is ‘True’ or ‘False’.
Python Hand-Written Notes
7 Question: – What are Loops in Python? Explain ‘for’ and ‘while’ Loop.
Loops are the programming constructs which is mainly used for repeatedly execute a block of code based on a condition.
For Loop : – For Loop is used for iterate over a sequence (such as lists, tuples, strings, or other iterable objects) or we also says that it execute a a code of block a specific number of time means it end when the final conditions match.
while Loop: – Continuously executes a block of code as long as specified condition is ‘True’. The loop continues until the condition becomes ‘False’.
8 Question: – How do you define a function in Python?
In Python, we define a function using the ‘def’ keyword followed by the function name and parentheses containing optional parameters. The basic syntax of defining a function in Python is:
def function_name(parameter1, parameter2, ...): # Function body - code block statement1 statement2 return value
9 Question: – Explain the difference between ‘return’ and ‘print’ in functions.
Here is difference between ‘return’ and ‘print’ in functions,
Characteristic | ‘return ‘ Statement | ‘print ‘ Statement |
---|---|---|
Purpose | Used to return a value from a function to its caller. | Used to display output on the console or terminal. |
Effect | Terminates the function and sends a value back to the caller. | Displays specified values or objects on the console. |
Usage | Typically used within a function to return a result or value. | Used to output intermediate or final results for debugging or user interaction. |
Output | Does not directly display anything on the console. | Directly displays specified values or objects on the console. |
Interaction | Enables communication between function and calling code by passing values. | Provides visual feedback during program execution. |
Handling Values | Can return any Python object (e.g., integers, strings, lists) as a result of function execution. | Only handles output for display purposes; does not pass values back to calling code. |
Example | def add(a, b): return a + b result = add(3, 5) print(result) Output: 8 | def greet(name): print(f”Hello, {name}!”) greet(“Alice”) Output: Hello, Alice! |
10 Question: – What are Lambda Function? Provide an example.
Lambda functions are also known as anonymous functions or lambda expressions, This is the concise way of defining small, one-line functions in Python without using the ‘def’ keyword. Lambda functions are typically used when you need a simple function for a short period of time, often as an argument to higher-order function like map(), filter(), or reduce().
Here is the example of Lambda Function,
add = lambda x, y: x + y result = add(3, 5) print(result) # Output: 8