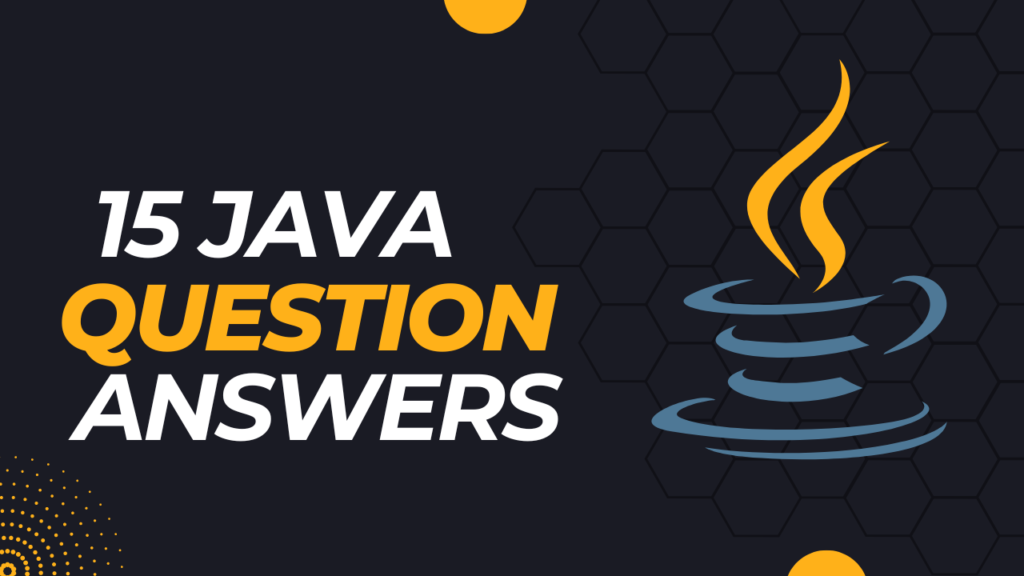
Question 1 : What is Java?
Java is a high-level and general purpose programming language which was developed by Sun Microsystems (now owned by Oracle Corporation) in the mid 1990s. It was designed for platform independent, meaning that java programs can run on any device that has a Java Virtual Machine (JVM) installed.
Question 2 : Features of Java?
Here are the main features of the Java,
- Platform-independent
- Object-Oriented
- Robust and Secure
- Multi-threaded
- Architecture-neutral
- Rich Standard library
- Dynamic and Extensible
Question 3 : How Java Platform Independent?
Java achieves platform independence through the following mechanisms:
- Bytecode
- Java virtual Machine
- Write Once, Run Anywhere (WORA)
Question 4 : Difference Between object and Classes.
- Class: – A class in Java is blueprint or template for creating objects. It is used for defines the attributes (fields) and behaviors (methods) that objects of the class will exhibit.
- Object: – An Object is an instance of the class. Which is used for represents a real-world entity and it is also used for creating “new” keyword followed by the class name. Objects have state (attributes) and behavior (methods) defined by their class.
Question 5 : Comments…
Comments in Java, like comments in most programming Language, do not show up in the executable program. Thus, you can add as many comments are needed without fear of bloating the code. Java has three way of making comments. the most common method is //. You use this for a comment that will run from the // to the end of the line.
When Longer comments are needed, you can mark each line with a //. Or you can use /* and */ comment delimiters that let you block off a longer comment.
Finally, a third kind of comment can be used to generate documentation automatically. This comment uses a /** to start and */ to end. For more on this type of comment and on automatic documentation generation.
Question 6 : About Data Types…
Java is strongly types language. This means that every variable must have a declared type. there are eight primitive types in java. Four of them are integer types; two are floating-point number types: one is character type char, used for code units in the Unicode encoding scheme; and one is a boolean type for truth values.
Question 7 : Integer Data Types…
The integer types are for numbers without fractional parts. Negative values are allowed. Java provides the four integer types which shown in table,
Type | Storage Requirement |
---|---|
int | 4 bytes |
short | 2 bytes |
long | 8 bytes |
byte | 1 byte |
Question 8 : Floating-Point Data Types…
The floating-point types denote numbers with fractional parts. The two floating-point types are shown in table,
Type | Storage Requirement |
---|---|
float | 4 bytes |
double | 8 bytes |
Question 9 : About Variables Declares in Java…
In java, every variable has a type. You declare a variable by placing the type first, following lowed by the name of the variable. Here are some exaple:
double salary;
int vacationDay;
long earthPopulation;
boolean done;
Question 10 : About Operators in Java…
The usual arithmetic operator + – * / are used in java for addition, subtraction, multiplication and division. The / operator denotes integer division if both arguments are integers and floating-point division otherwise. Integer remainder is denoted by %.
Question 11 : Strings…
Conceptually, Java Strings are sequences of Unicode characters. For example, the string “Java\u2122” consists of the five Unicode characters J, a, v, a, and TM. Java does not have a built-in string type. Instead, the standard Java library contains a predefined class called, naturally enough, String. Each quotes string is an instance of the String class.
Question 12 : Substrings…
You extract a substring from a larger string with the substring method of the String class. For example,
String greeting = "Hello";
String s = greeting.substring(0, 3);
creates a string consisting of the characters “Hel”.
Question 13 : Concatenation…
Java, like most programming languages, allow you to use the + sign to join (concatenate) two strings.
String expletive = "Expletive";
String PG13 = "deleted";
String message = expletive + PG13;
When you concatenate a string with a value that is not a string, the letter is converted to a string.
Question 14 : Code Points and Code Units
Java Strings are implemented as sequences of char values. Like the char data type is a code unit for representing Unicode code prints in the UTF-16 encoding. The most commonly used Unicode characters can be represented with a single code unit. The supplementary characters require a pair of code units.
Question 15 : The String API
The String class in java contains more than 50 methods. A surprisingly large number of them are sufficiently useful so that we can imagine using them frequently. – Each API note starts with the name of the class such as java.lang.String – the significance of the so-called package name java.lang. The class name is followed by the names, explanations, and parameters descriptions of one or more methods.