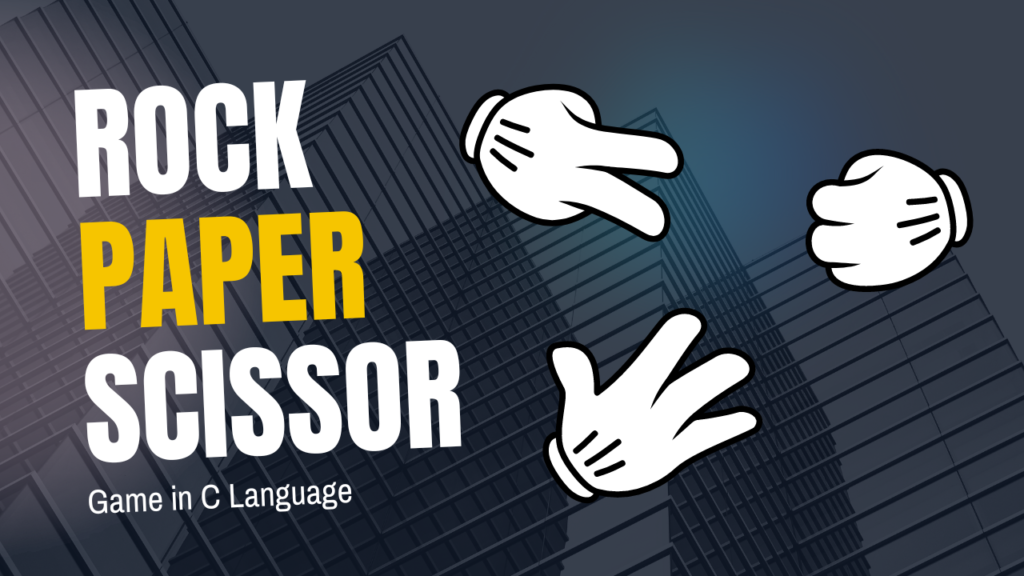
Table of Contents
Introduction – Rock, Paper & Scissor
In the Development of Game C has most important role, Many of the Popular games developed with C Language. So Here we create a Rock, Paper & Scissor Game.
Overview of the Game
Rock, Paper & Scissor Game is the simple but most popular hand game which is played between two peoples. Each player simultaneously forms one of three shapes using an outstretched hand: rock (a fist), paper (an open hand), or scissor (a fist with a index and middle fingers extended, forming a V). Here are the rules of game, which is pattern for creating a structure of the game.
- Rock Beats Scissor
- Scissor Beats Paper
- Paper Beats Rock
C Language Free Hand-Written Notes
Code Breakdown – Explanation
Here is Breakdown of Game codes Rock, Paper & Scissor For Explain how this game make:
Part 1 – Including Header Files
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
stdio.h
: This is a Standard input-output functions for console interaction.stdlib.h
: It is a Standard library functions likerand()
andsrand()
.time.h
: Here it Provides time-related functions for seeding the random number generator.
Part 2 – Function Definitons
1. ‘getUserChoice()’
int getUserChoice() {
int choice;
printf("Enter your choice:\n");
printf("1. Rock\n");
printf("2. Paper\n");
printf("3. Scissors\n");
scanf("%d", &choice);
return choice;
}
This Prompts is mainly used for the user to enter their choice (1 for Rock, 2 for Paper, 3 for Scissors) and returns the user’s input.
2. ‘getComputerChoice()’
int getComputerChoice() {
return rand() % 3 + 1; // Generates a random number between 1 and 3
}
Here this code Generates a random computer choice (Rock, Paper, or Scissors) using the rand()
function.
3. ‘determineWinner()’
void determineWinner(int userChoice, int computerChoice) {
if (userChoice == computerChoice) {
printf("It's a tie!\n");
} else if ((userChoice == 1 && computerChoice == 3) ||
(userChoice == 2 && computerChoice == 1) ||
(userChoice == 3 && computerChoice == 2)) {
printf("You win!\n");
} else {
printf("Computer wins!\n");
}
}
This Prompts is used for Compares the user’s choice with the computer’s choice to determine the winner based on the game rules.
Part 3 – Main Function
int main() {
int userChoice, computerChoice;
// Initialize random seed
srand(time(NULL));
printf("Welcome to Rock, Paper, Scissors!\n");
do {
userChoice = getUserChoice();
if (userChoice < 1 || userChoice > 3) {
printf("Invalid choice. Please enter a number between 1 and 3.\n");
continue;
}
computerChoice = getComputerChoice();
printf("You chose: ");
switch (userChoice) {
case 1:
printf("Rock\n");
break;
case 2:
printf("Paper\n");
break;
case 3:
printf("Scissors\n");
break;
}
printf("Computer chose: ");
switch (computerChoice) {
case 1:
printf("Rock\n");
break;
case 2:
printf("Paper\n");
break;
case 3:
printf("Scissors\n");
break;
}
determineWinner(userChoice, computerChoice);
// Ask if the user wants to play again
printf("Do you want to play again? (1 for Yes, 0 for No): ");
scanf("%d", &userChoice);
} while (userChoice == 1);
printf("Thanks for playing Rock, Paper, Scissors!\n");
return 0;
}
- Here the main() function which serves as the entry point of the program.
- Then, It initializes the random seed using srand(time(NULL)) to ensure different random numbers on each run.
- After showing each run, it Displays a welcome message and enters a loop where the game is played.
- Inside the loop, When the user’s choice is entered, the computer will choose its choice.
- After Choose of Choices, the winner is determined using determineWinner(), and the result will be displayed.
- The loop will continue until the user chooses not to play again.
Complete Project Codes
Here are the complete codes of the Project,
#include <stdio.h> #include <stdlib.h> #include <time.h> // Function to get user's choice int getUserChoice() { int choice; printf("Enter your choice:\n"); printf("1. Rock\n"); printf("2. Paper\n"); printf("3. Scissors\n"); scanf("%d", &choice); return choice; } // Function to generate computer's choice int getComputerChoice() { return rand() % 3 + 1; // Generates a random number between 1 and 3 } // Function to determine the winner void determineWinner(int userChoice, int computerChoice) { if (userChoice == computerChoice) { printf("It's a tie!\n"); } else if ((userChoice == 1 && computerChoice == 3) || (userChoice == 2 && computerChoice == 1) || (userChoice == 3 && computerChoice == 2)) { printf("You win!\n"); } else { printf("Computer wins!\n"); } } int main() { int userChoice, computerChoice; // Initialize random seed srand(time(NULL)); printf("Welcome to Rock, Paper, Scissors!\n"); do { userChoice = getUserChoice(); if (userChoice < 1 || userChoice > 3) { printf("Invalid choice. Please enter a number between 1 and 3.\n"); continue; } computerChoice = getComputerChoice(); printf("You chose: "); switch (userChoice) { case 1: printf("Rock\n"); break; case 2: printf("Paper\n"); break; case 3: printf("Scissors\n"); break; } printf("Computer chose: "); switch (computerChoice) { case 1: printf("Rock\n"); break; case 2: printf("Paper\n"); break; case 3: printf("Scissors\n"); break; } determineWinner(userChoice, computerChoice); // Ask if the user wants to play again printf("Do you want to play again? (1 for Yes, 0 for No): "); scanf("%d", &userChoice); } while (userChoice == 1); printf("Thanks for playing Rock, Paper, Scissors!\n"); return 0; }
Conclusion – Rock, Paper & Scissor
Here we create a Game of Rock, Paper & Scissor by the use of C Language. And explain the every possible and important Topic. Also gives here free Codes of the Game – So Enjoy it Guys…