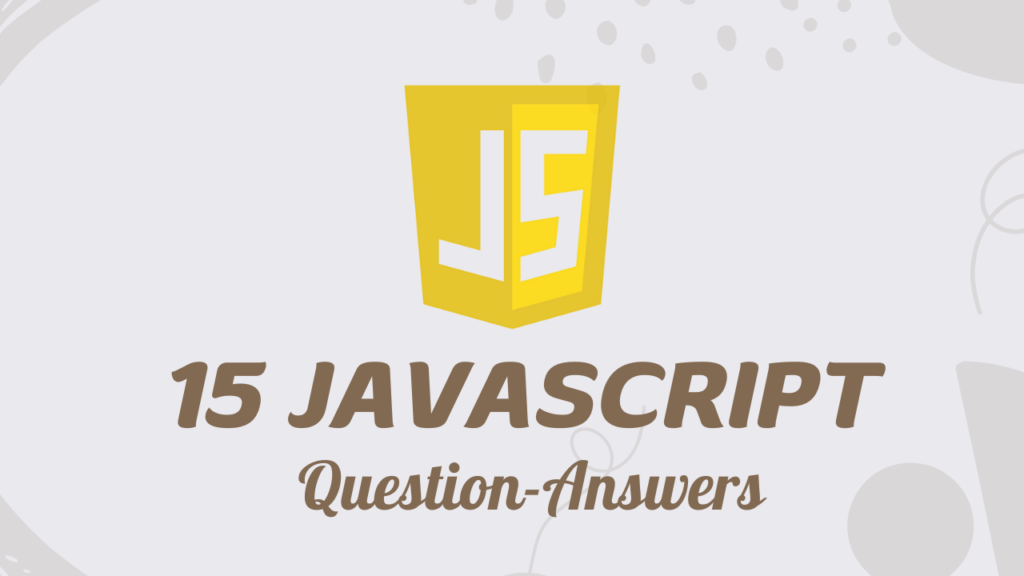
Ques 1 : What is JavaScript ?
JavaScript is an object-oriented programming language that allows creation of interactive Web pages. JavaScript allows user entries, which are loaded into an HTML form to be processed as required. This empowers a web site to return site information according to a user’s requests.
It Offers a several advantages to a Web Developer. A short development cycle. Ease of learning. Small size scripts. The strengths of JavaScript can be easily and quickly used to extend the functionality of HTML pages, already on a web site.
Ques 2 : Strings in Java Script ?
JavaScript Provides a built-in support for strings. A string is a sequence of zero or more characters that are enclosed by double (“) or single (‘) quotes. If a string begins with a double quote it must end with a double quote. If a string begins with a single quote it must end with a single quote.
Ques 3 : Null in Java Script ?
The null value is common to all JavaScript types. It is used to set a variable to an initial value that is different from other valid values. Use of the null value prevents the sort of errors that result from using uninitialized variables. The null value is automatically converted to default values of other types when used in an expression.
Ques 4 : Type Casting in Java Script ?
In JavaScript, variables are loosely cast. The type of a JavaScript variable is implicitly defined based on he literal values that are assigned to it from time to time.
For Instance, combining the string literal “Total amount is ” with the integer literal 1000 results in a string with the value “Total amount is 1000”. By contras, adding together the numeric literal 10.5 and string “20” results in the floating point integer literal 30.5. This process is known as Type Casting.
Ques 5 : Creating variables in Java Script ?
In order to make working with data types convenient, variables are created. In JavaScript variables can be created that can hold any type of data.
In order to use a variable, it is good programming style to declare it. Declaring a variable tells JavaScript that a variable of a given name exists so that the JavaScript interpreter can understand references to that variable name throughout the rest of the script. Variables can be declared using var command.
var <variable name> =value;
Ques 6 : The JavaScript Array…
Arrays are JavaScript objects that are capable of storing a sequence of values. These values are stored in indexed locations within the array. The length of an array is the number of elements that an array contains. The individual elements of an array are accessed by using the name of the array followed by the index value of the array element enclosed in square brackets.
Ques 7 : What is the Dense Array?
A Dense Array is an array that has been created with each of its elements being assigned a specific value. Dense arrays are used exactly in the same manner as other arrays. They are declared and initialized at the same time. Listings the values of the array elements in the array declaration creates dense arrays.
Ques 8 : Operators and Expression in JavaScript.
An Operator is used to transform one or more values into a single resultant value. the values to which the operator is applied is referred to as operands. A combinations of an operator and its operands is referred to as an expression.
Expressions are evaluated to determine the value of the expression. This is the value that results when an operator is applied to its operands.
Ques 9 : Condition Expression Ternary Operator.
JavaScript supports the conditional expression operator. They are ? and : The conditional expression operator is a ternary operator since it takes three operands, a condition to be evaluated and two alternative value to be returned based on the truth of falsity of the condition.
Ques 10 : Conditional Construct in JS.
The Conditional Construct in JavaScript offers a simple way to make a decision in a JavaScript program. The conditional construct will either return a “True” or “False” depending upon how the “condition” evaluated.
Ques 11 : Functions in JavaScript.
Function are block of JavaScript code that perform a specific task and often return a value. A JavaScript function may take zero or more parameters. Parameters are a standard technique via which control data can be passed to a function. Control data passed to a function, offers a means of controlling what a function returns.
Ques 12 : User-define Functions in JavaScript.
Functions offers the ability to group together JavaScript program code that performs a specific task into a single unit that can be used repeatedly whenever required in a JavaScript program.
A User-Defined function first needs to be declared and coded. Once this is done the function can be invoked by calling it using the name given to the function when it was declared. Functions can accepts the information in the form of arguments and can return results.
Ques 13 : Declaring Functions in JavaScript.
Functions are declared and created using the function keyword. A Function can comprise of the following:
- A name for the function
- A list of parameters (arguments) that will be that will accept values passed to the function when called.
- A block of JavaScript code that defines what the function does.
function function_name (parameter1, parameter2,...)
{
block of JavaScript Code
}
Ques 14 : Variable Scope in JS.
The parameters of a function are local to the function. They come into existence when the function is called and cease to exist when the function ends. For instance, in the example printName(), user exists only within the function printName() – it cannot be referred to or manipulated outside the function.
Also, any variable declared using var variable name within the function would have a scope limited to the function.
Ques 15 : Return Values in JS.
As with some JavaScript built-in functions, user defined functions can return values. Such values can be returned using the return statement. The return statement can be used to return any valid expression that evaluates to a single value.