C++ allows default arguments in functions to enhance flexibility, improve code readability, and reduce repetitive function overloading. Default arguments are values provided in a function declaration that are automatically used when no argument is passed for those parameters during a function call.
Why does C++ allow defaults arguments?
Simplifies Function Calls
By providing defaults values, a single function can handle multiple scenarios without requiring explicit arguments for each call. For example:
void greet(std::string name = "Guest") { std::cout << "Hello, " << name << "!\n"; } greet(); // Outputs: Hello, Guest! greet("Alice"); // Outputs: Hello, Alice!
Reduces Overloading
Instead of writing multiple overloaded functions, you can define a single function with default arguments, reducing redundancy and maintenance effort.
Improves Readability
Code becomes clearer as the intent of optional parameters is directly visible in the function signature.
YouTube
How are defaults arguments resolved?
Default arguments are resolved at compile time, not runtime. When a function is called without specifying some arguments, the compiler substitutes the missing arguments with their default values. For example:
void display(int x = 10, int y = 20) { std::cout << x << ", " << y << "\n"; } display(); // Compiler rewrites as display(10, 20) display(5); // Compiler rewrites as display(5, 20)
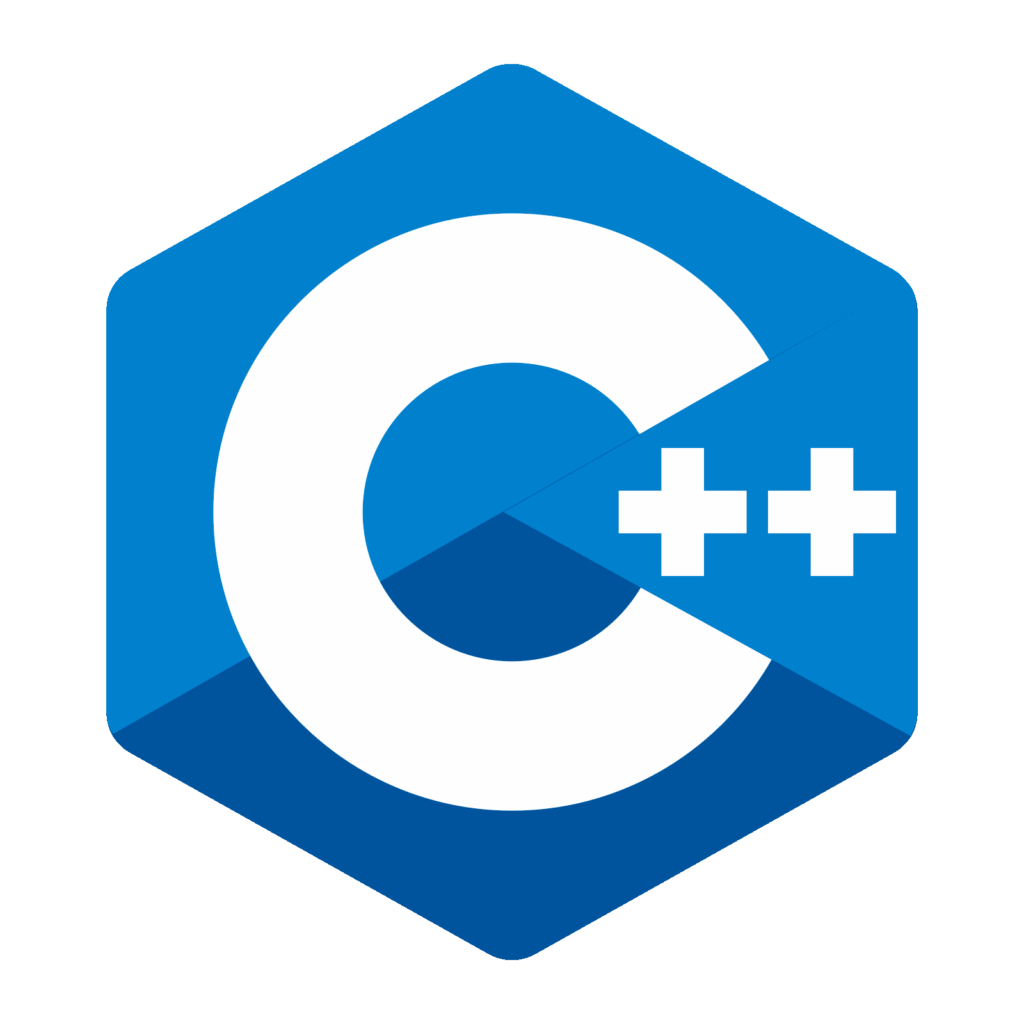